Class Analyze
First analysis
This command analyzes the dependencies between classes in a given path.
The <path>
argument is the path to the directory to analyze.
Note
To obtain accurate results, it is crucial to analyze your entire codebase. If you perform a partial analysis, some nodes may appear incomplete.
Here are the results obtained from the above command :
- Ac (Afferent Coupling) : number of classes that depend on the class.
- Ec (Efferent Coupling) : number of classes that the class depends on.
- I (Instability) : instability of the class.
- Na (Number of abstractions) : number of abstractions (interface, abstract class) contained in the class.
- A (Abstractness) : ratio of abstractions to the total number of methods in the class.
Class with a low instability (I close to 0) is strongly used and probably critical for the application, its business logic must be tested.
Class with a high instability (I close to 1) can suffer from side effects of its dependencies and must favor abstractions.
Class with a high abstractness (A close to 1) is totally abstract and should not have any concrete code.
The --human-readable
option allows you to obtain a more concise and human-readable result.
- Stability: from stable to unstable, with flexible as an intermediary.
- Abstractness: from abstract to concrete, with balanced as an intermediary.
A stable and concrete class is heavily used by the application and has few abstractions. It is probably not open to extension and it will be necessary to modify it to add behaviors. The modification of a stable class will impact other components of the application.
An unstable and concrete class will likely suffer from side effects caused by its dependencies, as its lack of abstraction leaves room for these side effects and makes the code harder to test.
Filtering results
To display only a list of namespaces, use the --only=
option.
To exclude a list of namespaces, use the --exclude=
option.
Graph rendering
You can render the graph of the analyzed classes using the --graph
option.
All the classes in the App\Application\Analyze
namespace are displayed :
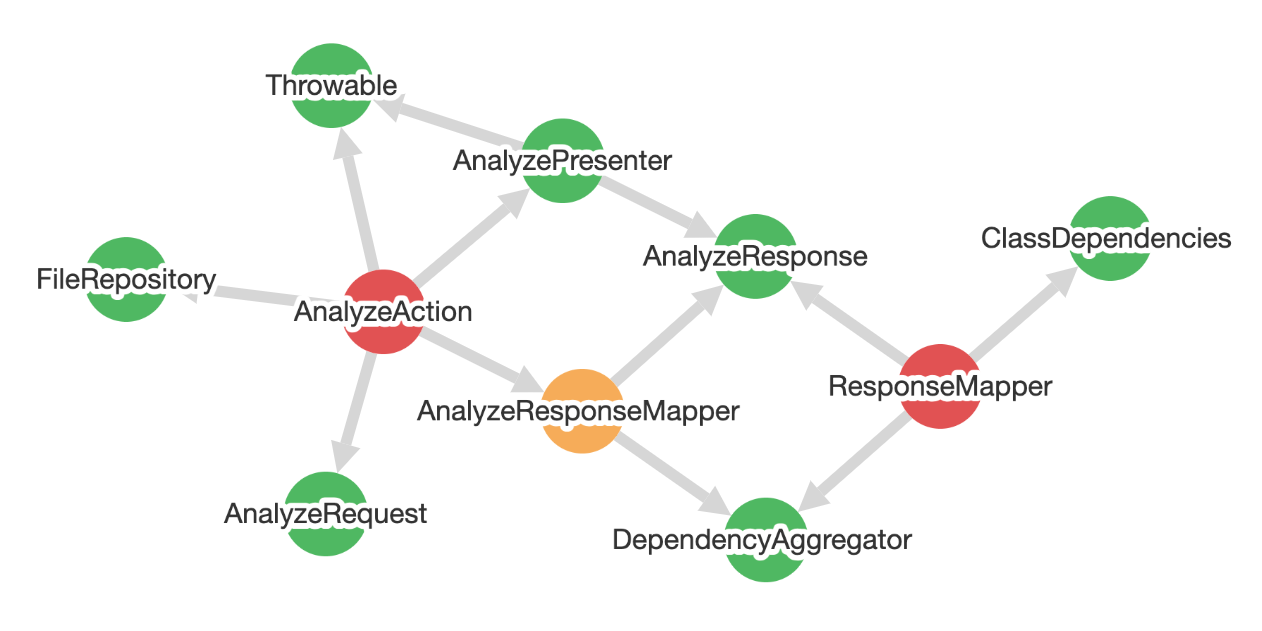
The color of the nodes indicates the stability of the class:
- A red node is strongly unstable.
- A yellow node is unstable.
- A green node is stable.
A red edge between two nodes indicates a weak dependency.
Note
This option generates a graph.html
file in the current directory.
Target class rendering
To render the graph of a specific class, use the --target=
option.
php class-dependencies-analyzer analyze:class app --target='App\Application\Analyze\AnalyzeAction' --graph
Note
It is necessary to analyze the entire codebase and use the --target=
option to target the class to render.
This will render the graph of all cascading dependencies of the target class.
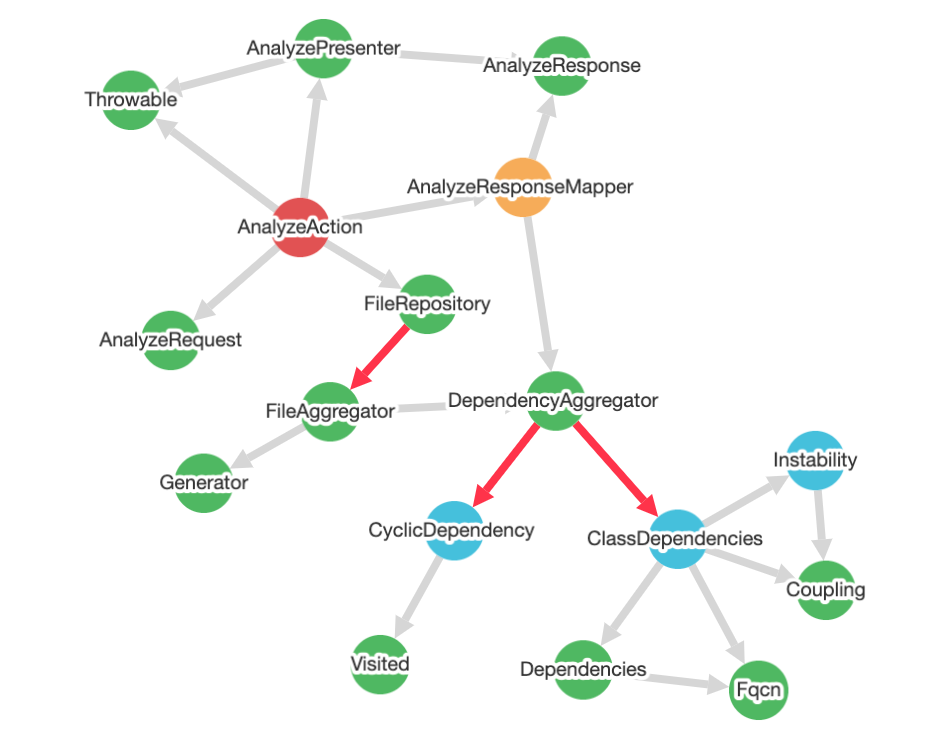
You can limit the depth of the target class dependencies using the --depth-limit=
option.
php class-dependencies-analyzer analyze:class app --target='App\Application\Analyze\AnalyzeAction' --graph --depth-limit=2
With a depth limit of 2, only the dependencies of the target class and its direct dependencies will be displayed.
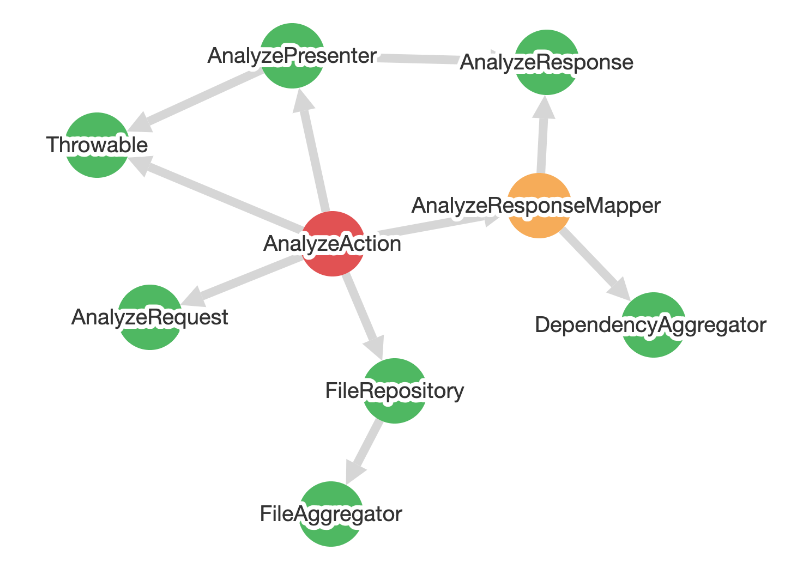